How to get the entity mapping to database table binding metadata from Hibernate
Imagine having a tool that can automatically detect JPA and Hibernate performance issues. Wouldn’t that be just awesome?
Well, Hypersistence Optimizer is that tool! And it works with Spring Boot, Spring Framework, Jakarta EE, Java EE, Quarkus, or Play Framework.
So, enjoy spending your time on the things you love rather than fixing performance issues in your production system on a Saturday night!
Introduction
Lukas Eder has posted a very interesting question on Stack Overflow, asking about a way of getting access to the binding metadata between entity mappings and database tables.
While I have previously answered a similar question using this article, I realized that particular use case was much simpler since it only extracted the database metadata. In this article, you are going to see how easily you can get the bridge information between your JPA entities and the underlying database schema.
How to get the entity mapping to database table binding metadata from Hibernate@vlad_mihalcea https://t.co/w972BC1ty0 pic.twitter.com/QXrrnYdOuK
— Java (@java) April 23, 2019
Integrator
Hibernate is very flexible, so it defines many SPI (Service Provider Interfaces) that you can register to customize Hibernate internals. One of these interfaces is org.hibernate.integrator.spi.Integrator
which is used by many technologies that integrate with Hibernate ORM, like Bean Validation, Envers or JACC Security Provider.
Using the Hibernate Integrator API, we can write our own component that captures the SessionFactory
build-time metadata which, otherwise, is only available during bootstrap.
public class MetadataExtractorIntegrator implements org.hibernate.integrator.spi.Integrator { public static final MetadataExtractorIntegrator INSTANCE = new MetadataExtractorIntegrator(); private Database database; private Metadata metadata; public Database getDatabase() { return database; } public Metadata getMetadata() { return metadata; } @Override public void integrate( Metadata metadata, SessionFactoryImplementor sessionFactory, SessionFactoryServiceRegistry serviceRegistry) { this.database = metadata.getDatabase(); this.metadata = metadata; } @Override public void disintegrate( SessionFactoryImplementor sessionFactory, SessionFactoryServiceRegistry serviceRegistry) { } }
The org.hibernate.boot.Metadata
is what we are interested in since it contains the PersistentClass
entity bindings.
To register MetadataExtractorIntegrator
with Hibernate we have two possibilities based on the underlying bootstrap method.
Hibernate-native boostrap
If you’re using the Hibernate-native bootstrap, then you can register the Integrator
with the BootstrapServiceRegistryBuilder
as follows:
final BootstrapServiceRegistry bootstrapServiceRegistry = new BootstrapServiceRegistryBuilder() .enableAutoClose() .applyIntegrator( MetadataExtractorIntegrator.INSTANCE ) .build(); final StandardServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder(bootstrapServiceRegistry) .applySettings(properties()) .build();
JPA boostrap
If you’re using the JPA bootstrap, then you can register the Integrator
with the BootstrapServiceRegistryBuilder
as follows:
Map<String, Object> configuration = new HashMap<>(); Integrator integrator = integrator(); if (integrator != null) { configuration.put("hibernate.integrator_provider", (IntegratorProvider) () -> Collections.singletonList( MetadataExtractorIntegrator.INSTANCE ) ); } EntityManagerFactory entityManagerFactory = new EntityManagerFactoryBuilderImpl( new PersistenceUnitInfoDescriptor(persistenceUnitInfo), configuration ) .build();
That’s for when you want to bootstrap JPA without any persistence.xml
configuration file.
To see how you can set the
hibernate.integrator_provider
configuration property when using Spring with JPA or Spring with Hibernate, check out this article.
Domain Model
Assuming we have the following database tables mapped by our JPA application:
When running the following test case:
Metadata metadata = MetadataExtractorIntegrator.INSTANCE.getMetadata(); for ( PersistentClass persistentClass : metadata.getEntityBindings()) { Table table = persistentClass.getTable(); LOGGER.info( "Entity: {} is mapped to table: {}", persistentClass.getClassName(), table.getName() ); for(Iterator propertyIterator = persistentClass.getPropertyIterator(); propertyIterator.hasNext(); ) { Property property = (Property) propertyIterator.next(); for(Iterator columnIterator = property.getColumnIterator(); columnIterator.hasNext(); ) { Column column = (Column) columnIterator.next(); LOGGER.info( "Property: {} is mapped on table column: {} of type: {}", property.getName(), column.getName(), column.getSqlType() ); } } }
Hibernate generates the following output:
Entity: com.vladmihalcea.book.hpjp.util.providers.entity.BlogEntityProvider$Tag is mapped to table: tag Property: name is mapped on table column: name of type: varchar(255) Property: version is mapped on table column: version of type: integer Entity: com.vladmihalcea.book.hpjp.util.providers.entity.BlogEntityProvider$PostComment is mapped to table: post_comment Property: post is mapped on table column: post_id of type: bigint Property: review is mapped on table column: review of type: varchar(255) Property: version is mapped on table column: version of type: integer Entity: com.vladmihalcea.book.hpjp.util.providers.entity.BlogEntityProvider$Post is mapped to table: post Property: title is mapped on table column: title of type: varchar(255) Property: version is mapped on table column: version of type: integer Entity: com.vladmihalcea.book.hpjp.util.providers.entity.BlogEntityProvider$PostDetails is mapped to table: post_details Property: createdBy is mapped on table column: created_by of type: varchar(255) Property: createdOn is mapped on table column: created_on of type: datetime(6) Property: version is mapped on table column: version of type: integer
I'm running an online workshop on the 20-21 and 23-24 of November about High-Performance Java Persistence.
If you enjoyed this article, I bet you are going to love my Book and Video Courses as well.
Conclusion
Hibernate is highly customizable, and the Integrator
SPI allows you to get access to the Hibernate Metadata
which you can later inspect from your enterprise application.
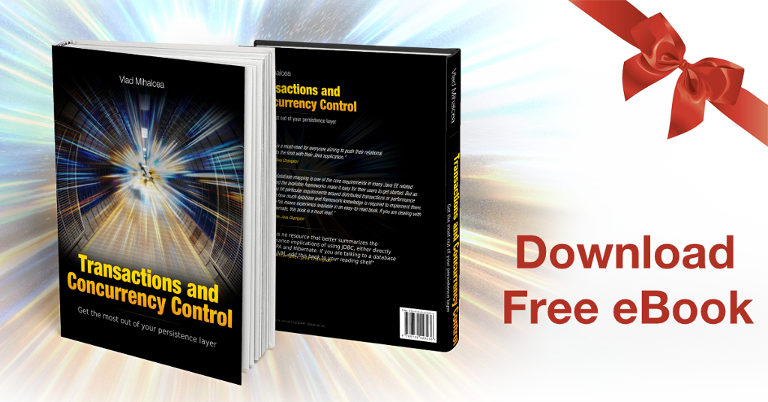